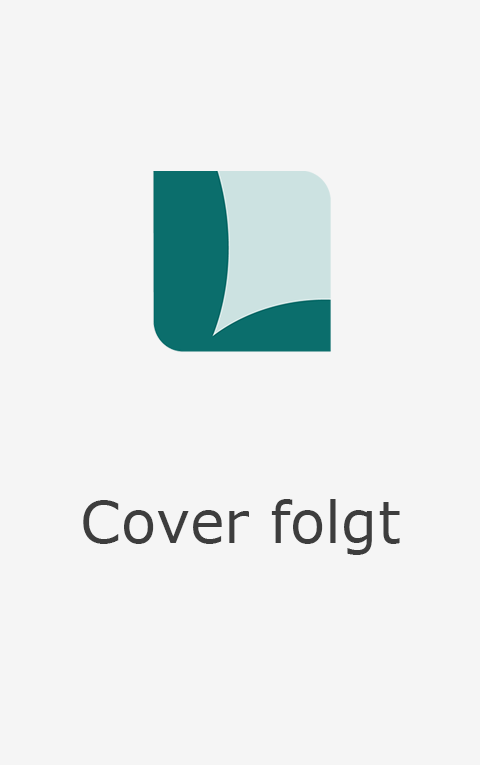
Data Abstraction and Problem Solving with Java
Pearson (Verlag)
978-0-321-30428-5 (ISBN)
- Titel erscheint in neuer Auflage
- Artikel merken
PART ONE - Problem-Solving Techniques
1 Review of Java Fundamentals
1.1 Program Structure
1.2 Language Basics
1.3 Selection Statements
1.4 Iteration Statements
1.5 Useful Java Classes
1.6 Java Exceptions
1.7 Text Input and Output
1.8 File Input and Output
2 Principles of Programming and Software Engineering
2.1 Problem Solving and Software Engineering
2.2 Achieving an Object-Oriented Design
2.3 A Summary of Key Issues in Programming
3 Recursion: The Mirrors
3.1 Recursive Solutions
3.2 Counting Things
3.3 Searching an Array
3.4 Organizing Data
3.5 Recursion and Efficiency
4 Data Abstraction: The Walls
4.1 Abstract Data Types
4.2 Specifying ADTs
4.3 Implementing ADTs
5 Linked Lists
5.1 Preliminaries
5.2 Programming with Linked Lists
5.3 Variations of the Linked List
5.4 Application: Maintaining an Inventory
5.5 The Java Collections Framework
PART TWO - Problem Solving with Abstract Data Types
6 Recursion as a Problem-Solving Technique
6.1 Backtracking
6.2 Defining Languages
6.3 The Relationship Between Recursion and Mathematical Induction
7 Stacks
7.1 The Abstract Data Type Stack
7.2 Simple Applications of the ADT Stack
7.3 Implementations of the ADT Stack
7.4 Application: Algebraic Expressions
7.5 Application: A Search Problem
7.6 The Relationship Between Stacks and Recursion
8 Queues
8.1 The Abstract Data Type Queue
8.2 Simple Applications of the ADT Queue
8.3 Implementations of the ADT Queue
8.4 A Summary of Position-Oriented ADTs
8.5 Application: Simulation
9 Advanced Java Topics
9.1 Inheritance Revisited
9.2 Dynamic Binding and Abstract Classes
9.3 The ADTs List and Sorted List Revisited
9.4 Java Generics
9.5 Iterators
10 Algorithm Efficiency and Sorting
10.1 Measuring the Efficiency of Algorithms
10.2 Sorting Algorithms and Their EfficiencySelection Sort
11 Trees
11.1 Terminology
11.2 The ADT Binary Tree
11.3 The ADT Binary Search Tree
11.4 General Trees
12 Tables and Priority Queues
12.1 The ADT Table
12.2 The ADT Priority Queue: A Variation of the ADT Table
12.3 Tables and Priority Queues in the JCF
13 Advanced Implementations of Tables
13.1 Balanced Search Trees
13.2 Hashing
13.3 Data with Multiple Organizations
14 Graphs
14.1 Terminology
14.2 Graphs as ADTs
14.3 Graph Traversals
14.4 Applications of Graphs
15 External Methods
15.1 A Look at External Storage
15.2 Sorting Data in an External File
15.3 External Tables
APPENDICES
A A Comparison of Java to C++
B Unicode Character Codes (ASCII Subset)
C Java Resources
D Mathematical Induction 828
Glossary
Answers to Self-Test Exercises
Index
Erscheint lt. Verlag | 25.11.2005 |
---|---|
Sprache | englisch |
Maße | 231 x 189 mm |
Gewicht | 1292 g |
Themenwelt | Informatik ► Programmiersprachen / -werkzeuge ► Java |
Mathematik / Informatik ► Informatik ► Web / Internet | |
ISBN-10 | 0-321-30428-4 / 0321304284 |
ISBN-13 | 978-0-321-30428-5 / 9780321304285 |
Zustand | Neuware |
Haben Sie eine Frage zum Produkt? |
aus dem Bereich