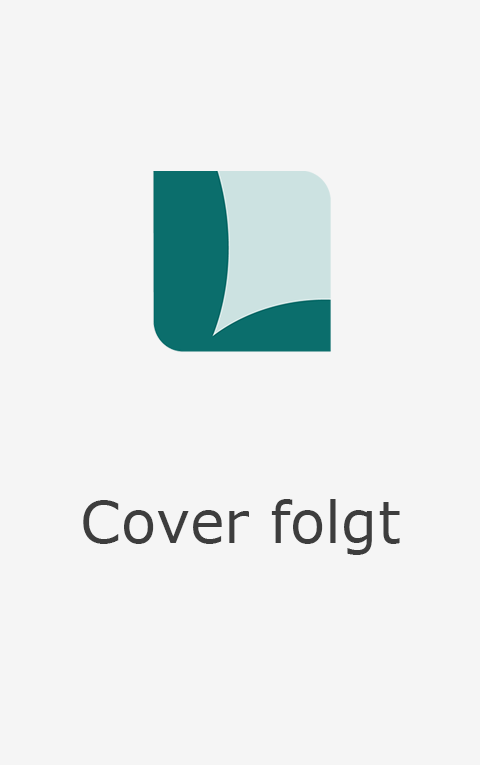
Professional C# and .NET
Wrox Press (Verlag)
978-1-119-79720-3 (ISBN)
In Professional C# and .NET: 2021 Edition, Microsoft MVP for Visual Studio and Development Technologies and veteran developer, Christian Nagel, delivers a comprehensive tour of the new features and capabilities of C#9 and .NET 5.
Experienced programmers making the transition to C# will benefit from the author’s in-depth explorations to create Web- and Windows applications using ASP.NET Core, Blazor, and WinUI using modern application patterns and new features offered by .NET including Microservices deployed to Docker images, GRPC, localization, asynchronous streaming, and much more.
The book also offers:
Discussions of the extension of .NET to non-Microsoft platforms like OSX and Linux
Explanations of the newest features in C#9, including support for record types, and enhanced support for tuples, pattern matching, and nullable reference types
Integrating .NET applications with Microsoft Azure services such as Azure App
Configuration, Azure Key Vault, Azure Functions, the Azure Active Directory, and others Downloadable code examples from wrox.com and github.com with online updates for C# 10 and .NET 6
Perfect for programmers with a background in C#, Visual Basic, Java, or C/C++, Professional C# and .NET: 2021 Edition will also earn a place in the libraries of software architects seeking an up-to-date and fulsome treatment of the latest C# and .NET releases.
Christian Nagel is a Microsoft MVP for Visual Studio and Development Technologies, software architect, and veteran developer who has been building solutions with .NET technologies since 2000. He has authored many acclaimed .NET books, and he also speaks at such international conferences as Ignite (formerly TechEd) and Tech Days. A supporter of .NET user groups, Christian is a Microsoft Certified Trainer and Professional Developer for WinUI and .NET MAUI Apps, ASP.NET Core, and Microsoft Azure.
Introduction xxxix
Part I: The C# Language
Chapter 1: .NET Applications and Tools 3
From .NET Framework to .NET Core to .NET 3
.NET Terms 4
.NET Support Length 9
Application Types and Technologies 10
Microsoft Azure 12
Developer Tools 14
Using the .NET CLI 16
Summary 23
Chapter 2: Core C# 24
Fundamentals of C# 25
Nullable Types 30
Using Predefined Types 33
Controlling Program Flow 37
Organization with Namespaces 44
Working with Strings 45
Comments 49
C# Preprocessor Directives 51
C# Programming Guidelines 54
Summary 58
Chapter 3: Classes, Records, Structs, and Tuples 59
Creating and Using Types 60
Pass by Value or by Reference 60
Classes 62
Records 77
Structs 79
Enum Types 80
ref, in, and out 83
Tuples 86
ValueTuple 88
Deconstruction 88
Pattern Matching 89
Partial Types 92
Summary 94
Chapter 4: Object-Oriented Programming in C# 95
Object Orientation 96
Inheritance with Classes 96
Modifiers 104
Inheritance with Records 106
Using Interfaces 107
Generics 115
Summary 118
Chapter 5: Operators and Casts 119
Operators 120
Using Binary Operators 127
Type Safety 132
Operator Overloading 136
Comparing Objects for Equality 139
Implementing Custom Indexers 142
User-Defined Conversions 143
Summary 152
Chapter 6: Arrays 153
Multiple Objects of the Same Type 154
Simple Arrays 154
Multidimensional Arrays 157
Jagged Arrays 158
Array Class 159
Arrays as Parameters 163
Enumerators 163
Using Span with Arrays 167
Indices and Ranges 170
Array Pools 172
BitArray 174
Summary 176
Chapter 7: Delegates, Lambdas, and Events 177
Referencing Methods 178
Delegates 178
Lambda Expressions 187
Events 189
Summary 192
Chapter 8: Collections 193
Overview 194
Collection Interfaces and Types 194
Lists 195
Stacks 206
Linked Lists 208
Sorted List 209
Dictionaries 211
Sets 218
Performance 220
Immutable Collections 222
Summary 225
Chapter 9: Language Integrated Query 226
LINQ Overview 227
Standard Query Operators 233
Parallel LINQ 256
Expression Trees 258
LINQ Providers 261
Summary 262
Chapter 10: Errors and Exceptions 263
Handling Errors 264
Predefined Exception Classes 264
Catching Exceptions 265
User-Defined Exception Classes 278
Caller Information 285
Summary 287
Chapter 11: Tasks and Asynchronous Programming 288
Why Asynchronous Programming Is Important 289
Task-Based Async Pattern 290
Tasks 291
Error Handling 297
Cancellation of async Methods 299
Async Streams 300
Async with Windows Apps 302
Summary 306
Chapter 12: Reflection, Metadata, And Source Generators 307
Inspecting Code at Runtime and Dynamic Programming 308
Custom Attributes 308
Using Reflection 314
Using Dynamic Language Extensions for Reflection 322
ExpandoObject 325
Source Generators 327
Summary 334
Chapter 13: Managed and Unmanaged Memory 335
Memory 336
Memory Management Under the Hood 336
Strong and Weak References 342
Working with Unmanaged Resources 344
Unsafe Code 349
Span 365
Platform Invoke 368
Summary 373
Part II: Libraries
Chapter 14: Libraries, Assemblies, Packages, and Nuget 377
The Hell of Libraries 378
Assemblies 379
Creating and Using Libraries 381
Creating NuGet Packages 386
Module Initializers 390
Summary 391
Chapter 15: Dependency Injection and Configuration 392
What Is Dependency Injection? 393
Using the .NET DI Container 393
Using the Host Class 395
Lifetime of Services 396
Initialization of Services Using Options 403
Using Configuration Files 405
Configuration with .NET Applications 406
Azure App Configuration 411
Summary 418
Chapter 16: Diagnostics and Metrics 419
Diagnostics Overview 420
Logging 421
Metrics 429
Analytics with Visual Studio App Center 434
Application Insights 437
Summary 439
Chapter 17: Parallel Programming 440
Overview 441
Parallel Class 442
Tasks 448
Cancellation Framework 455
Channels 458
Timers 461
Threading Issues 463
Interlocked 468
Monitor 468
SpinLock 469
WaitHandle 470
Mutex 470
Semaphore 471
Events 473
Barrier 476
ReaderWriterLockSlim 479
Locks with await 481
Summary 484
Chapter 18: Files and Streams 485
Overview 486
Managing the File System 486
Iterating Files 492
Working with Streams 493
Using Readers and Writers 503
Compressing Files 505
Watching File Changes 508
JSON Serialization 509
Using Files and Streams with the Windows Runtime 515
Summary 519
Chapter 19: Networking 520
Overview 521
Working with Utility Classes 521
Using Sockets 526
Using TCP Classes 533
Using UDP 537
Using Web Servers 542
The HttpClient Class 548
HttpClient Factory 554
Summary 557
Chapter 20: Security 558
Elements of Security 559
Verifying User Information 559
Encrypting Data 566
Ensuring Web Security 576
Summary 581
Chapter 21: Entity Framework Core 582
Introducing EF Core 583
Creating a Model 593
Scaffolding a Model from the Database 600
Migrations 601
Working with Queries 606
Loading Related Data 612
Working with Relationships 617
Saving Data 625
Conflict Handling 630
Using Transactions 635
Using Azure Cosmos DB 639
Summary 643
Chapter 22: Localization 644
Global Markets 645
Namespace System.Globalization 645
Resources 656
Localization with ASP.NET Core 658
Localization with WinUI 664
Summary 667
Chapter 23: Tests 668
Overview 668
Unit Testing 669
Using a Mocking Library 678
ASP.NET Core Integration Testing 682
Summary 684
Part III: Web Applications and Services
Chapter 24: Asp.NET Core 687
Understanding Web Technologies 687
Creating an ASP.NET Core Web Project 689
Adding Client-Side Content 694
Creating Custom Middleware 696
Endpoint Routing 699
Request and Response 700
Session State 706
Health Checks 708
Deployment 711
Summary 713
Chapter 25: Services 714
Understanding Today’s Services 715
REST Services with ASP.NET Core 715
Creating a .NET Client 724
Using EF Core with Services 730
Authentication and Authorization with Azure AD B2C 732
Implementing and Using Services with GRPC 740
Using Azure Functions 748
More Azure Services 751
Summary 751
Chapter 26: Razor Pages and MVC 752
Setting Up Services for Razor Pages and MVC 753
Razor Pages 755
ASP.NET Core MVC 773
Summary 778
Chapter 27: Blazor 779
Blazor Server and Blazor WebAssembly 780
Creating a Blazor Server Web Application 782
Blazor WebAssembly 788
Razor Components 792
Summary 800
Chapter 28: Signalr 801
Overview 801
Creating a Simple Chat Using SignalR 802
Grouping Connections 810
Streaming with SignalR 814
Summary 816
Part IV: Apps
Chapter 29: Windows Apps 819
Introducing Windows Apps 819
Introducing XAML 826
Working with Controls 837
Working with Data Binding 852
Implementing Navigation 861
Implementing Layout Panels 867
Summary 875
Chapter 30: Patterns with XAML Apps 876
Why MVVM? 876
Defining the MVVM Pattern 877
Sample Solution 879
Models 879
Services 882
View Models 883
Views 891
Messaging Using Events 897
Summary 898
Chapter 31: Styling Windows Apps 899
Styling 900
Shapes 900
Geometry 902
Transformation 903
Brushes 906
Styles and Resources 908
Templates 913
Animations 918
Visual State Manager 928
Summary 931
Index 933
Erscheinungsdatum | 24.11.2021 |
---|---|
Sprache | englisch |
Maße | 188 x 234 mm |
Gewicht | 1656 g |
Themenwelt | Mathematik / Informatik ► Informatik |
ISBN-10 | 1-119-79720-9 / 1119797209 |
ISBN-13 | 978-1-119-79720-3 / 9781119797203 |
Zustand | Neuware |
Haben Sie eine Frage zum Produkt? |
aus dem Bereich