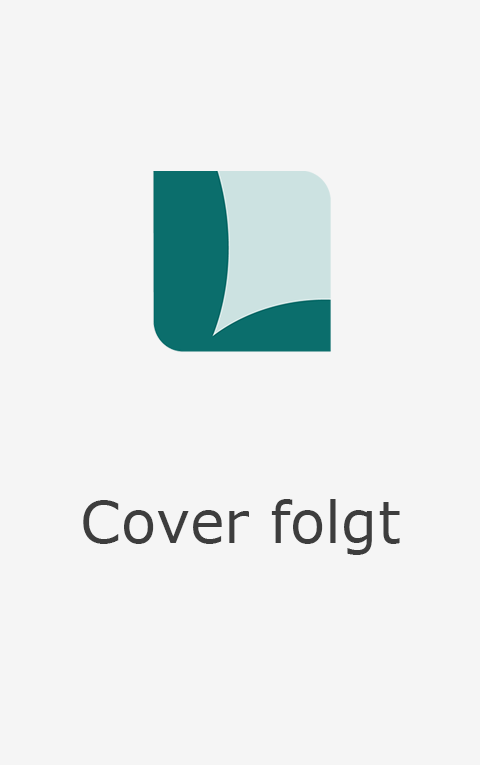
Object-Oriented Computation in C++ and Java
Addison Wesley (Hersteller)
978-0-13-348861-6 (ISBN)
- Keine Verlagsinformationen verfügbar
- Artikel merken
Virtually all business, scientific, and engineering applications are heavily reliant on numeric data items. C++ and Java offer object-oriented programmers unique flexibility and control over the computations required within such applications.
However, most books on object-oriented programming gloss over such numeric data items, emphasizing instead one-dimensional containers or collections and components of the graphical user interface.
Object-Oriented Computation in C++ and Java fills the gap left by such books.
Drawing on more than twenty years' experience as a software developer, tester, consultant, and professor, Conrad Weisert shows readers how to use numeric objects effectively.
Not limited to any language or methodology, the concepts and techniques discussed in this book are entirely independent of one's choice of design and coding methodology.
Practitioners of Extreme Programming, UML-driven design, agile methods, incremental development, and so on will all develop these same data classes.
Whether you are a seasoned professional or an advanced computer science student, this book can teach you techniques that will improve the quality of your programming and the efficiency of your applications. The exercises (and answers) presented in this book with teach you new ways to implement the computational power of C++, Java, and numeric data items.
Topics include
taxonomy of data types
developing and using object-oriented classes for numeric data
design patterns for commonly occurring numeric data types
families of interacting numeric data types
choosing efficient and flexible internal data representations
techniques for exploiting pattern reuse in C++
conventions for arithmetic operations in Java
numeric vectors and matrices
CONRAD WEISERT is a leader and innovator in applying systematic approaches to information system design and large-scale project management. Before establishing Information Disciplines, Inc., a Chicago consulting firm, he was responsible for data-center operations, application system development, professional staff training, and technical services at numerous large organizations. Through his firm, he consults to banks, insurance companies, manufacturers, local government agencies, and computer service firms, to demonstrate the effectiveness of modern, enlightened methods in producing high-quality results. He has been active as a member of and speaker at various professional organizations for more than twenty years, and now, in addition to training done through his consulting practice, teaches computer science courses at Loyola University.
&>
Preface
Introduction 3
Chapter 1: Numeric Objects in Context 7
1.1 Data and objects 7
1.2 Application-domain data 8
1.3 Non-application-domain data 9
1.4 Four basic types of elementary data 10
1.5 Avoiding false composites 12
1.6 Numeric data representation 12
Chapter 2: Review of C++ and Java Facilities and Techniques for Defining Classes 21
2.1 Our emphasis 21
2.2 The basic goal-a major difference between C++ and Java 22
2.3 Constructors and destructor 24
2.4 Operator overloading in C++ 31
2.5 Operator overloading in Java 37
2.6 Flow-control constructs 38
2.7 Manipulating character strings in C++ 41
2.8 Canonical class structure 43
2.9 Overcoming macrophobia 44
2.10 Program readability 48
2.11 Error detection and exceptions 53
Chapter 3: Defining a Pure Numeric Data Type 55
3.1 What does "pure numeric" mean? 55
3.2 Example: Designing a Complex number class 55
3.3 Packaging and using the Complex class 65
3.4 Some other pure numeric classes 67
3.5 Java equivalents 70
Chapter 4: Defining a Numeric Type Having an Additive
4.1 Unit of measure in modeling real-world data 73
4.2 A business application example: Money class 75
4.3 Noting the additive pattern 83
4.4 Supporting an external Money representation 88
4.5 More additive classes 93
4.6 Additive classes in Java 96
Chapter 5: The Point-Extent Pattern for Pairs of Numeric Types 100
5.1 Non-additive numeric types 100
5.2 Another companion class: Calendarlnfo 106
5.3 Back to Date and Days 109
5.4 Other Point-Extent pairs 119
5.5 Date and Days classes in Java 121
5.6 Other point-extent classes in Java 124
Chapter 6: Families of Interacting Numeric Types 125
6.1 Beyond the patterns 125
6.2 Example: Electrical circuit quantities 126
6.3 Greater interaction: Newton's laws in a straight line .... 134
6.4 Extending Newtonian classes to three-dimensional space 140
6.5 Other families of interacting types 145
6.6 Summary 146
6.7 Java versions 146
Chapter 7: Role of Inheritance and Polymorphism with Numeric Types 148
7.1 Review of example classes 148
7.2 Representation is not specialization 149
7.3 Usage is not specialization 150
7.4 A numeric specialization example 151
7.5 Obstacles to polymorphic functions 153
7.6 Turning off Java polymorphism 154
7.7 Why bother with OOP? 154
Chapter 8: Programming with Numeric Vectors and Matrices 156
8.1 Introduction 156
8.2 Existing facilities 156
8.3 A C++ base class for all arrays 160
8.4 Some specialized vector classes 164
8.5 Operations on numeric arrays 166
8.6 A basic Matrix class 171
8.7 Some specialized Matrix classes 174
8.8 What about Java? 177
Appendix: Answers to Selected Exercises 179
Index 191
Reihe/Serie | Dorset House eBooks |
---|---|
Verlagsort | Boston |
Sprache | englisch |
Gewicht | 1 g |
Themenwelt | Informatik ► Software Entwicklung ► Objektorientierung |
ISBN-10 | 0-13-348861-6 / 0133488616 |
ISBN-13 | 978-0-13-348861-6 / 9780133488616 |
Zustand | Neuware |
Haben Sie eine Frage zum Produkt? |