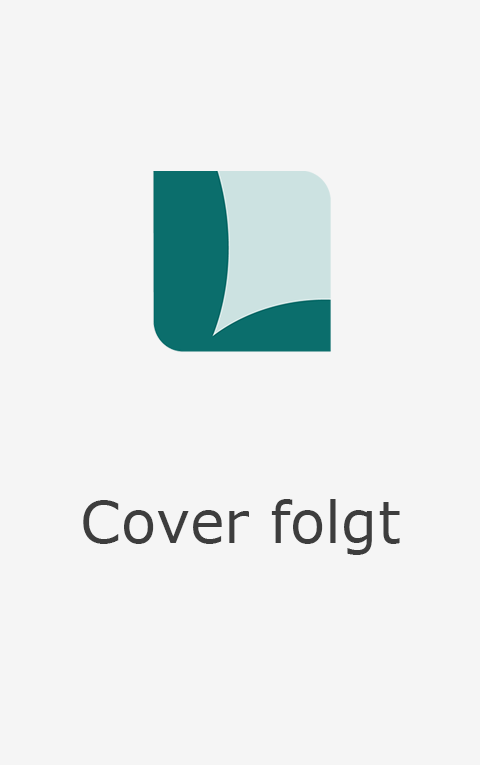
Big Java
John Wiley & Sons Inc (Verlag)
978-1-119-39872-1 (ISBN)
- Titel ist leider vergriffen;
keine Neuauflage - Artikel merken
Choosing the enhanced eText format allows students to develop their coding skills using targeted, progressive interactivities designed to integrate with the eText. All sections include built-in activities, open-ended review exercises, programming exercises, and projects to help students practice programming and build confidence. These activities go far beyond simplistic multiple-choice questions and animations. They have been designed to guide students along a learning path for mastering the complexities of programming. Students demonstrate comprehension of programming structures, then practice programming with simple steps in scaffolded settings, and finally write complete, automatically graded programs.
The perpetual access VitalSource Enhanced eText, when integrated with your school’s learning management system, provides the capability to monitor student progress in VitalSource SCORECenter and track grades for homework or participation.
*Enhanced eText and interactive functionality available through select vendors and may require LMS integration approval for SCORECenter.
Preface iii
Special Features xxiv
1 Introduction 1
1.1 Computer Programs 2
1.2 The Anatomy of a Computer 3
1.3 The Java Programming Language 6
1.4 Becoming Familiar with Your Programming Environment 7
1.5 Analyzing Your First Program 11
1.6 Errors 14
1.7 PROBLEM SOLVING Algorithm Design 15
2 Fundamental Data Types 31
2.1 Variables 32
2.2 Arithmetic 43
2.3 Input and Output 50
2.4 PROBLEM SOLVING First Do it By Hand 59
2.5 Strings 61
3 Decisions 83
3.1 The if Statement 84
3.2 Comparing Numbers and Strings 90
3.3 Multiple Alternatives 98
3.4 Nested Branches 102
3.5 PROBLEM SOLVING Flowcharts 107
3.6 PROBLEM SOLVING Test Cases 110
3.7 Boolean Variables and Operators 113
3.8 APPLICATION Input Validation 118
4 Loops 142
4.1 The while Loop 142
4.2 PROBLEM SOLVING Hand-Tracing 149
4.3 The for Loop 152
4.4 The do Loop 158
4.5 APPLICATION Processing Sentinel Values 160
4.6 PROBLEM SOLVING Storyboards 164
4.7 Common Loop Algorithms 167
4.8 Nested Loops 174
4.9 PROBLEM SOLVING Solve a Simpler Problem First 178
4.10 APPLICATION Random Numbers and Simulations 182
5 Methods 211
5.1 Methods as Black Boxes 212
5.2 Implementing Methods 214
5.3 Parameter Passing 217
5.4 Return Values 220
5.5 Methods Without Return Values 224
5.6 PROBLEM SOLVING Reusable Methods 225
5.7 PROBLEM SOLVING Stepwise Refinement 229
5.8 Variable Scope 236
5.9 Recursive Methods (Optional) 240
6 Arrays and Arraylists 261
6.1 Arrays 262
6.2 The Enhanced for Loop 269
6.3 Common Array Algorithms 270
6.4 Using Arrays with Methods 280
6.5 PROBLEM SOLVING Adapting Algorithms 284
6.6 PROBLEM SOLVING Discovering Algorithms by Manipulating Physical Objects 291
6.7 Two-Dimensional Arrays 294
6.8 Array Lists 301
7 Input/Output and Exception Handling 331
7.1 Reading and Writing Text Files 332
7.2 Text Input and Output 337
7.3 Command Line Arguments 345
7.4 Exception Handling 352
7.5 APPLICATION Handling Input Errors 361
8 Objects and Classes 375
8.1 Object-Oriented Programming 376
8.2 Implementing a Simple Class 378
8.3 Specifying the Public Interface of a Class 381
8.4 Designing the Data Representation 385
8.5 Implementing Instance Methods 386
8.6 Constructors 389
8.7 Testing a Class 393
8.8 Problem Solving: Tracing Objects 399
8.9 Object References 403
8.10 Static Variables and Methods 408
8.11 PROBLEM SOLVING Patterns for Object Data 410
8.12 Packages 417
9 Inheritance and Interfaces 437
9.1 Inheritance Hierarchies 438
9.2 Implementing Subclasses 442
9.3 Overriding Methods 446
9.4 Polymorphism 452
9.5 Object: The Cosmic Superclass 463
9.6 Interface Types 470
10 Graphical User Interfaces 493
10.1 Frame Windows 494
10.2 Events and Event Handling 498
10.3 Processing Text Input 509
10.4 Creating Drawings 515
11 Advanced User Interfaces 535
11.1 Layout Management 536
11.2 Choices 538
11.3 Menus 549
11.4 Exploring the Swing Documentation 556
11.5 Using Timer Events for Animations 561
11.6 Mouse Events 564
12 Object-Oriented Design 577
12.1 Classes and Their Responsibilities 578
12.2 Relationships Between Classes 582
12.3 APPLICATION Printing an Invoice 589
13 Recursion 607
13.1 Triangle Numbers 608
13.2 Recursive Helper Methods 616
13.3 The Efficiency of Recursion 618
13.4 Permutations 623
13.5 Mutual Recursion 628
13.6 Backtracking 634
14 Sorting and Searching 649
14.1 Selection Sort 650
14.2 Profiling the Selection Sort Algorithm 653
14.3 Analyzing the Performance of the Selection Sort Algorithm 656
14.4 Merge Sort 661
14.5 Analyzing the Merge Sort Algorithm 664
14.6 Searching 668
14.7 PROBLEM SOLVING Estimating the Running Time of an Algorithm 673
14.8 Sorting and Searching in the Java
15 The Java Collections Framework 691
15.1 An Overview of the Collections Framework 692
15.2 Linked Lists 695
15.3 Sets 701
15.4 Maps 706
15.5 Stacks, Queues, and Priority Queues 712
15.6 Stack and Queue Applications 715
16 Basic Data Structures 735
16.1 Implementing Linked Lists 736
16.2 Implementing Array Lists 751
16.3 Implementing Stacks and Queues 755
16.4 Implementing a Hash Table 761
17 Tree Structures 779
17.1 Basic Tree Concepts 780
17.2 Binary Trees 784
17.3 Binary Search Trees 789
17.4 Tree Traversal 798
17.5 Red-Black Trees 804
17.6 Heaps 811
17.7 The Heapsort Algorithm 822
18 Generic Classes 837
18.1 Generic Classes and Type Parameters 838
18.2 Implementing Generic Types 839
18.3 Generic Methods 843
18.4 Constraining Type Parameters 845
18.5 Type Erasure 849
19 Stream Processing 859
19.1 The Stream Concept 860
19.2 Producing Streams 862
19.3 Collecting Results 864
19.4 Transforming Streams 866
19.5 Lambda Expressions 869
19.6 The Optional Type 873
19.7 Other Terminal Operations 876
19.8 Primitive-Type Streams 877
19.9 Grouping Results 880
19.10 Common Algorithms Revisited 882
20 Advanced Input/Output 897
20.1 Readers, Writers, and Input/Output Streams 898
20.2 Binary Input and Output 899
20.3 Random Access 903
20.4 Object Input and Output Streams 908
20.5 File and Directory Operations 913
21 Multithreading (Web Only)
21.1 Running Threads
21.2 Terminating Threads
21.3 Race Conditions
21.4 Synchronizing Object Access
21.5 Avoiding Deadlocks
21.6 APPLICATION Algorithm Animation
22 Internet Networking (Web Only)
22.1 The Internet Protocol
22.2 Application Level Protocols
22.3 A Client Program
22.4 A Server Program
22.5 URL Connections
23 Relational Databases (Web Only)
23.1 Organizing Database Information
23.2 Queries
23.3 Installing a Database
23.4 Database Programming in Java
23.5 APPLICATION Entering an Invoice
ST 2 Transactions
ST 3 Object-Relational Mapping
WE 1 Programming a Bank Database
24 XML (WEB ONLY)
24.1 XML Tags and Documents
24.2 Parsing XML Documents
24.3 Creating XML Documents
24.4 Validating XML Documents
25 Web Applications (WEB ONLY)
25.1 The Architecture of a Web Application
25.2 The Architecture of a JSF Application
25.3 JavaBeans Components
25.4 Navigation Between Pages
25.5 JSF Components
25.6 APPLICATION A Three-Tier Application
Appendix A The Basic Latin and Latin-1 Subsets of Unicode A-1
Appendix B Java Operator Summary A-5
Appendix C Java Reserved Word Summary A-7
Appendix D The Java Library A-9
Appendix E Java Language Coding Guidelines A-38
Appendix F Tool Summary
Appendix G Number Systems
Appendix H UML Summary
Appendix I Java Syntax Summary
Appendix J HTML Summary
Glossary G-1
Index I-1
Credits C-1
Erscheinungsdatum | 25.11.2021 |
---|---|
Verlagsort | New York |
Sprache | englisch |
Maße | 203 x 257 mm |
Gewicht | 1724 g |
Themenwelt | Informatik ► Programmiersprachen / -werkzeuge ► Java |
Mathematik / Informatik ► Informatik ► Web / Internet | |
ISBN-10 | 1-119-39872-X / 111939872X |
ISBN-13 | 978-1-119-39872-1 / 9781119398721 |
Zustand | Neuware |
Informationen gemäß Produktsicherheitsverordnung (GPSR) | |
Haben Sie eine Frage zum Produkt? |